Action Types
Text¶
Extract JSON¶
This action automatically converts all JSON properties/fields to Variables.
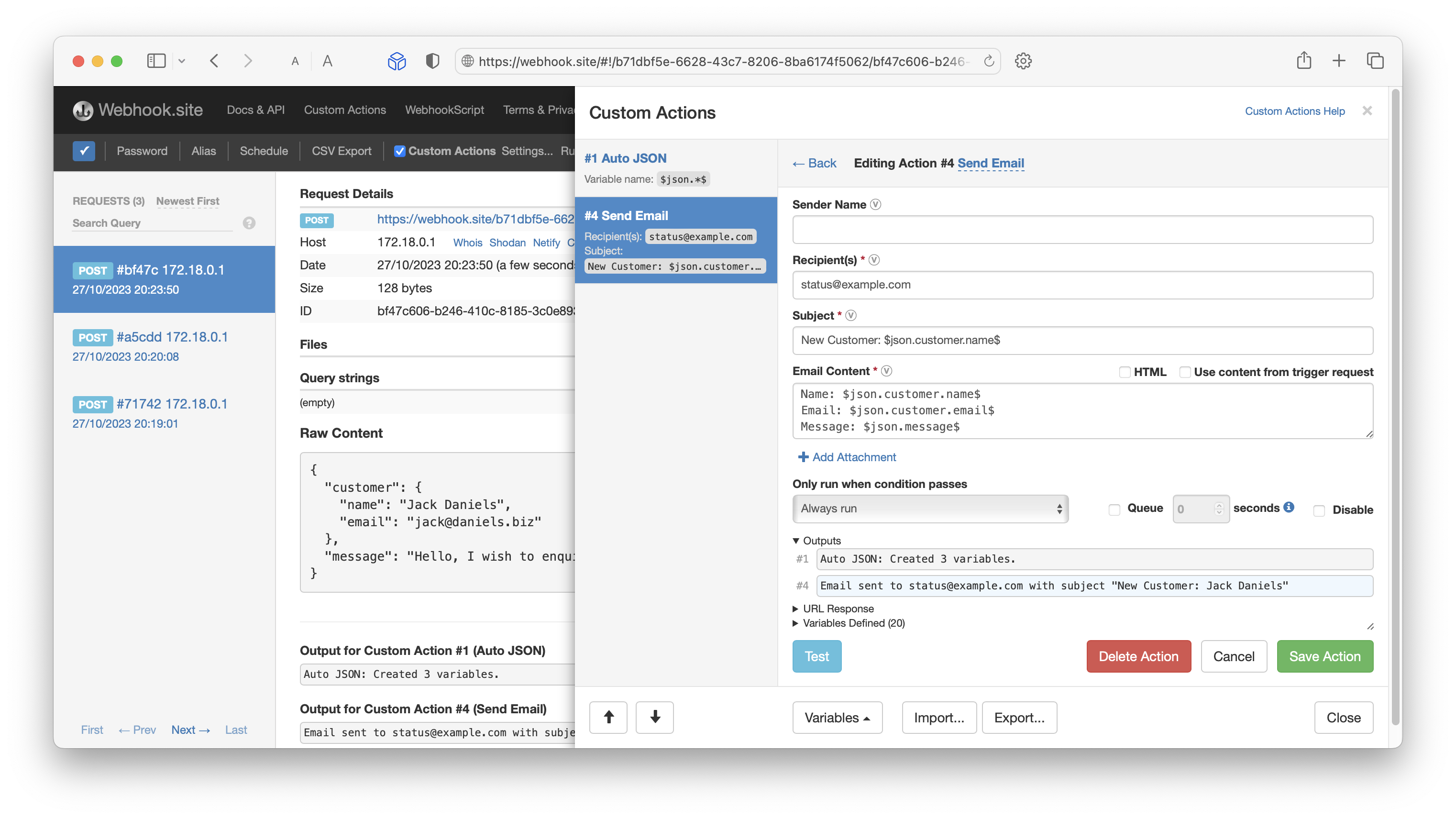
Per default, the action works on the JSON found in the $request.content$
variable, e.g. the request body data.
If the JSONPath parameter is specified, this can be used to limit the variable creation to only the subset of data specified by the JSONPath query.
Extract JSON Example¶
If the following data is specified in the Source parameter:
{
"Actors": [
{
"name": "Tom Cruise",
"age": 56,
"Born At": "Syracuse, NY",
"Birthdate": "July 3, 1962",
"photo": "https://jsonformatter.org/img/tom-cruise.jpg"
},
{
"name": "Robert Downey Jr.",
"age": 53,
"Born At": "New York City, NY",
"Birthdate": "April 4, 1965",
"photo": "https://jsonformatter.org/img/Robert-Downey-Jr.jpg"
}
]
}
If the JSONPath parameter is empty, the following 10 variables will be created:
Variable Name | Value |
---|---|
$json.Actors.0.name$ |
Tom Cruise |
$json.Actors.0.age$ |
56 |
$json.Actors.0.Born At$ |
Syracuse, NY |
$json.Actors.0.Birthdate$ |
July 3, 1962 |
$json.Actors.0.photo$ |
https://example.com/tom-cruise.jpg |
$json.Actors.1.name$ |
Robert Downey Jr. |
$json.Actors.1.age$ |
53 |
$json.Actors.1.Born At$ |
New York City, NY |
$json.Actors.1.Birthdate$ |
April 4, 1965 |
$json.Actors.1.photo$ |
https://example.com/Robert-Downey-Jr.jpg |
If the JSONPath parameter is set to .Actors.0
, only the following 5 variables are created:
Variable Name | Value |
---|---|
$json.0.name$ |
Tom Cruise |
$json.0.age$ |
56 |
$json.0.Born At$ |
Syracuse, NY |
$json.0.Birthdate$ |
July 3, 1962 |
$json.0.photo$ |
https://example.com/tom-cruise.jpg |
Extract JSONPath¶
Warning
This action has been deprecated and can no longer be chosen. We recommend using the Extract JSON action as it supports creating multiple variables in one go, saving you from creating duplicate actions.
This action runs a JSONPath query on the contents of a request. With it, you can extract any data from a JSON document and store it in a variable, which can then be used in a downstream action.
JSONPath is very similar to the jq
commandline utility.
JSONPath Examples¶
Example data:
{
"store": {
"name": "Cool Books Ltd",
"books": [
{
"title": "12 Rules for Life",
"author": "Jordan B. Peterson",
"author.age": 60,
"price": 10,
"isbn": "13123123123"
},
{
"title": "How to Win Friends and Influence People",
"author": "Dale Carnegie",
"price": 9,
"isbn": "23482394"
}
]
}
}
JSONPath | Result |
---|---|
.store.name |
name property of store object |
.store.books[0]["author.age"] |
author age of first book (bracket syntax can be useful for e.g. keys containing periods) |
$.store.books[*].author |
the authors of all books in the store |
$..author |
all authors |
$.store..price |
the price of everything in the store. |
$..books[2] |
the third book |
$..books[(@.length-1)] |
the last book in order. |
$..books[-1:] |
the last book in order. |
$..books[0,1] |
the first two books |
$..books[:2] |
the first two books |
$..books[::2] |
every second book starting from first one |
$..books[1:6:3] |
every third book starting from 1 till 6 |
$..books[?(@.isbn)] |
filter all books with isbn number property |
$..books[?(@.isbn != '')] |
filter all books with isbn that isn't null or "" (empty string.) (Bracket syntax can also be used here, e.g. .values[?(@['my value'] != '')] ) |
$..books[?(@.price<10)] |
filter all books cheaper than 10 |
$..* |
all elements in the data (recursively extracted) |
JSONPath Syntax¶
Symbol | Description |
---|---|
$ |
The root object/element (not strictly necessary) |
@ |
The current object/element |
. or [] |
Child operator |
.. |
Recursive descent |
* |
Wildcard. All child elements regardless their index. |
[,] |
Array indices as a set |
[start:end:step] |
Array slice operator borrowed from ES4/Python. |
?() |
Filters a result set by a script expression |
() |
Uses the result of a "script" expression as the index |
For more details on what's possible with JSONPath, take a look at the docs.
As you start entering a JSONPath, the results are validated and shown next to the input field.
Validate JSON¶
Allows validating that a given input is valid, parsable JSON – optionally also against a JSON Schema.
When the JSON Schema is a URL, the schema is downloaded from the server.
The action generates 2 variables:
$validator.count$
- the count of errors$validator.errors$
– the errors in JSON format
If the JSON is invalid or does not conform to the schema, an Action error is generated.
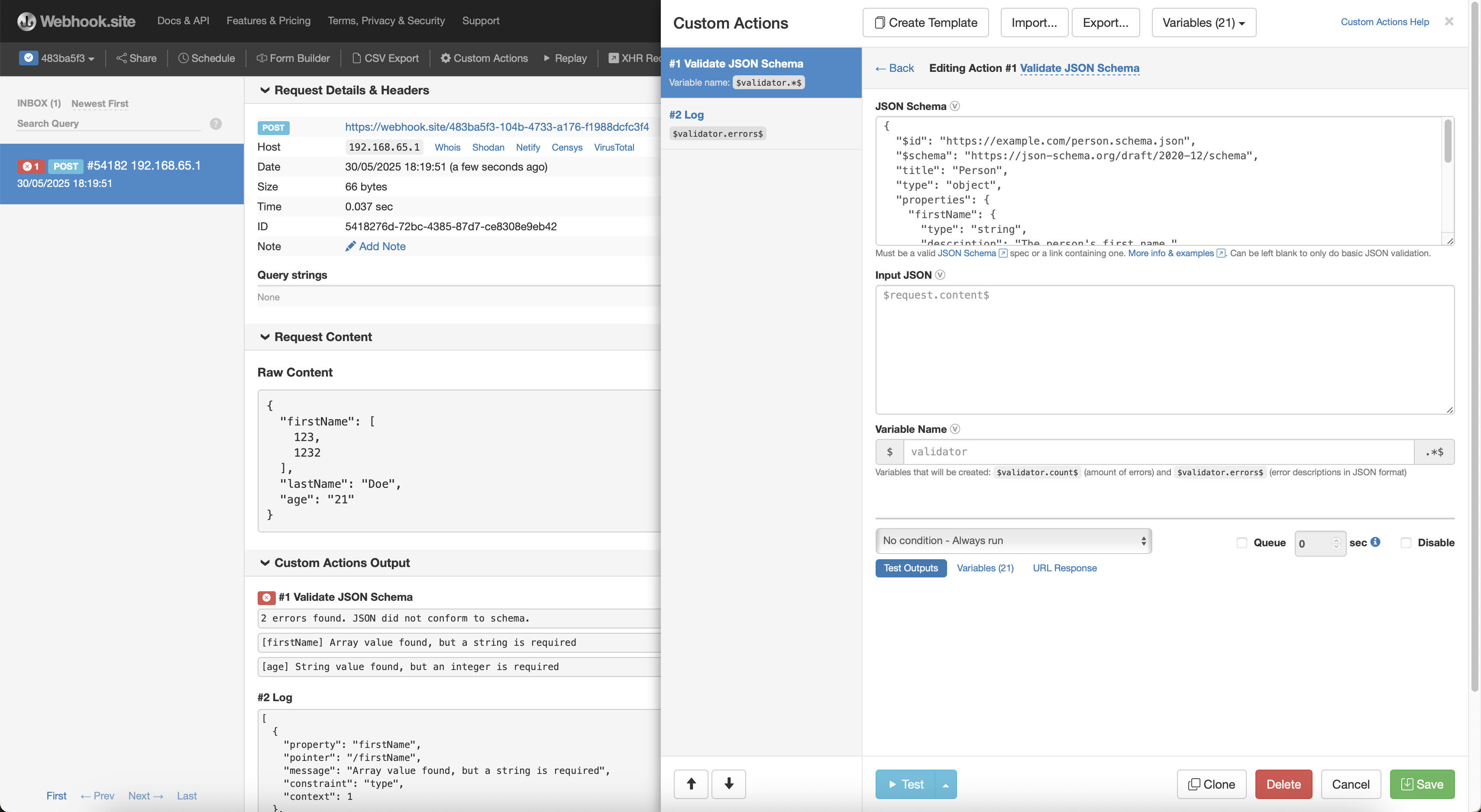
Example JSON Schema¶
{
"$id": "https://example.com/person.schema.json",
"$schema": "https://json-schema.org/draft/2020-12/schema",
"title": "Person",
"type": "object",
"properties": {
"firstName": {
"type": "string",
"description": "The person's first name."
},
"lastName": {
"type": "string",
"description": "The person's last name."
},
"age": {
"description": "Age in years which must be equal to or greater than zero.",
"type": "integer",
"minimum": 0
}
}
}
The following JSON will match and cause $validator.count$
to be 0
:
However, if the following JSON is specified:
Then $validator.count$
would be set to 2
, and $validator.errors$
would contain:
[
{
"property": "firstName",
"pointer": "/firstName",
"message": "Array value found, but a string is required",
"constraint": "type",
"context": 1
},
{
"property": "age",
"pointer": "/age",
"message": "String value found, but an integer is required",
"constraint": "type",
"context": 1
}
]
Extract Regex¶
This action runs a Regex (regular expression) query on the contents of a request. With it, you can extract any data from a text document and store it in a variable, which can then be used in a downstream action.
As you start entering a Regex, the results are validated and shown next to the input field.
Extract XPath¶
Similar to the Extract JSONPath Custom Action, Extract XPath lets you extract values from an XML or HTML document and save the result as a variable.
XPath Examples¶
The following examples are based on this XML document:
<?xml version="1.0"?>
<organization name="ExampleCo">
<employees>
<employee id="1">Jack</employee>
<employee id="2">Ann</employee>
</employees>
</organization>
Example XPath | Notes | Result |
---|---|---|
/organization |
Finds all content within the organization element | Jack Ann |
//employee[@id != 1] |
// traverses all <employee> elements in document, the @id query selects all except those with id =1 |
Jack |
/organization/@name |
@name to get the "name" property of the element |
ExampleCo |
/organization/employees/employee[2] |
[2] specifies 2nd element |
Ann |
/organization/employees/employee[2]/@id |
Get the "id" property of second employee element | 2 |
/organization/employees/employee[@id=1] |
Employee element with id property equal to "1" | Jack |
/organization/employees/employee[last()] |
Last employee element | Ann |
//employee[contains(@id, "2")] |
Employee within any parent element where id contains "2" | Ann |
For more examples, see W3CSchools or XPath Cheatsheet
Replace Text¶
An action that allows replacing multiple inputs to a string with specified replacements. Additionally, Webhook.site will replace all variables in the source text as well as the text being replaced, and the replacement.
Split Text¶
Split text into multiple variables. Using hello,world
as Source, and ,
as Delimiter, 2 variables will be created: $variable.1$
is "hello" and $variable.2$
is "world".
Entering \n
as Delimiter will split text by each line.
The maximum amount of variables created is 1024.
Map Text¶
Sets a variable depending on what maps to the source value and operator.
If we set Source to John
, Operator to ends with
, Variable Name to $user_id$
, Default to unknown
, and add a mapping of From: John
-> To: 123
, then the variable name $user_id$
would be set to 123
.
If the Source had been Jack
, $user_id$
would have been set to unknown.
Network¶
HTTP Request¶
This will send a HTTP/HTTPS request from the Webhook.site cloud.
The HTTP Request action has several modes:
- Text: In the default text mode, this allows sending plain-text content, but also data like JSON and XML.
- JSON: Similar to the Text mode, but also sets the Content-Type header to application/json and allows JSON formatting.
- Multipart: With Multipart selected, it is possble to build a form/multipart request and send form data and files. Note that the Filename and Content-Type fields are not required.
- URL Encoded: In URL Encoded mode, the keys and values are sent using URL Encoding.
- Forward: In forward mode, all data sent to the Webhook.site URL is forwarded here, including the HTTP method, query strings, headers and body data. It is possible to overwrite the method and append headers. To forward the HTTP method, set the Method dropdown to the blank option.
The response of the request is stored in a series of variable names prefixed with a value of your choosing. The following variables are set after the request has been fired:
$your_prefix.content$
- response body content$your_prefix.status$
- response status code$your_prefix.headers$
- response headers$your_prefix.url$
- the URL the request was sent to$your_prefix.error$
- if the request resulted in an error, it's stored in this variable.
The maximum timeout for a HTTP request is 15 seconds when the action is run during the request. When the action is in Queue mode, the maximum timeout is raised to 60 seconds.
Retry¶
When checked, this option will cause the HTTP to be retried in case of a network error, timeout, or related issue.
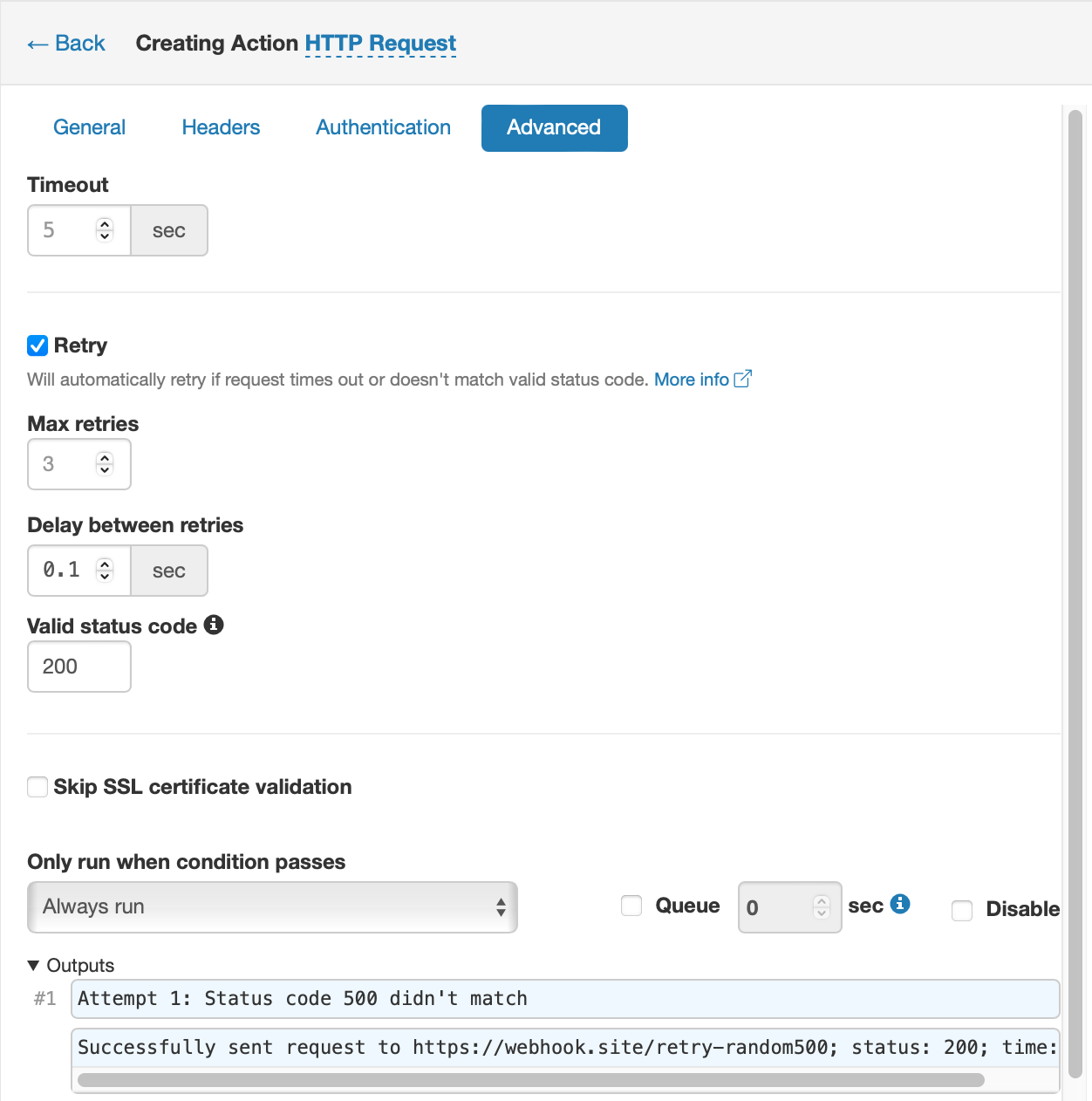
Per default, there are 3 retries (4 requests total) with 1 second delay between each retry.
Note
As Webhook.site URLs have a maximum of 30 seconds to respond, it's best to use a low delay and retry number that stays under 30 seconds total. Otherwise there's a risk the actions won't complete. Alternatively, you can mark the HTTP Request action as Queued, which has a total maximum runtime of 120 seconds.
If a status code is specified, the request will also be retried when the response status doesn't match the specified status code. The character *
can be used as wildcard, e.g. 20*
will match any status code in the range 200-209.
Each retry attempt will add an extra line of output.
Send Email¶
This will send a email with variable contents from the Webhook.site cloud. Variables extracted previously can be used.
Send Email (SMTP)¶
This will send a email with variable contents from your own email provider. Variables extracted previously can be used.
Gmail¶
For Gmail, the following specific setup is required:
- Hostname:
smtp.gmail.com
- Port:
587
- Username: youraccount@gmail.com or youraccount@example.com (replace with your Gmail address)
- Password: You must create a Mail App Password
- Encryption:
TLS
As of 1 May 2025, this method is no longer available to Google Workspace customers. We recommend using the Webhook.site Custom Domain to send emails from your own domain.
SSH¶
We recommend authenticating using a pre-generated keypair, which can be created under Control Panel → Providers.
All SSH-based actions have a max timeout of 30 seconds.
Run SSH Command¶
Allows you to run one or more SSH command on a server. Webhook.site captures the output (stdout), stderr and the command exit code as Variables that can be used in downstream actions:
$ssh.stdout$
$ssh.stderr$
$ssh.exit$
SFTP Upload¶
Allows uploading a file to a SFTP (SSH) server, specifying a hostname, port, username, password, relative path to the file. The file content can be specified, in which Variables are replaced.
SFTP Download¶
Allows downloading a file to a SFTP (SSH) server, specifying a hostname, port, username, password and the path to the file. The file content is downloaded to a Variable.
FTP(S) Upload¶
Allows uploading a file to a FTP or FTPS (FTP with TLS/SSL) server, specifying a hostname, port, username, password, relative path to the file, whether to use SSL and whether to use passive mode. Finally, the file content can be specified, in which Variables are replaced.
We recommend storing the password as a Global Variable.
FTP(S) Download¶
Allows downloading a file to a FTP or FTPS (FTP with TLS/SSL) server, specifying a hostname, port, username, password, path, whether to use SSL and whether to use passive mode. The file content is downloaded to a Variable.
We recommend storing the password as a Global Variable.
Database Query¶
Allows running a database query, with support for fetching out data in a series of variables. We recommend storing the password as a Global Variable. The query timeout is 10 seconds.
Supported Database Servers¶
Currently supported are:
- PostgreSQL
- MySQL/MariaDB
- Microsoft SQL Server
If your database server is not on the list, please contact support.
Using Parameters¶
When using e.g. INSERT or UPDATE statements, you should use parameters for each column value. Doing this, you avoid SQL injection attacks and other issues when using user-submitted data (e.g. via Variables), or even just data containing special characters like quotes, that could otherwise break a query.
Each parameter name should start with a colon (:) and be a single word. You can then reference these parameters inside the query, like in the following example:
You can auto-create the parameters from the statement by clicking the Fill in Parameters from Statement button. This works with both positional (?, ?)
and named (:id, :value)
parameters. You cannot mix named and positional parameters.
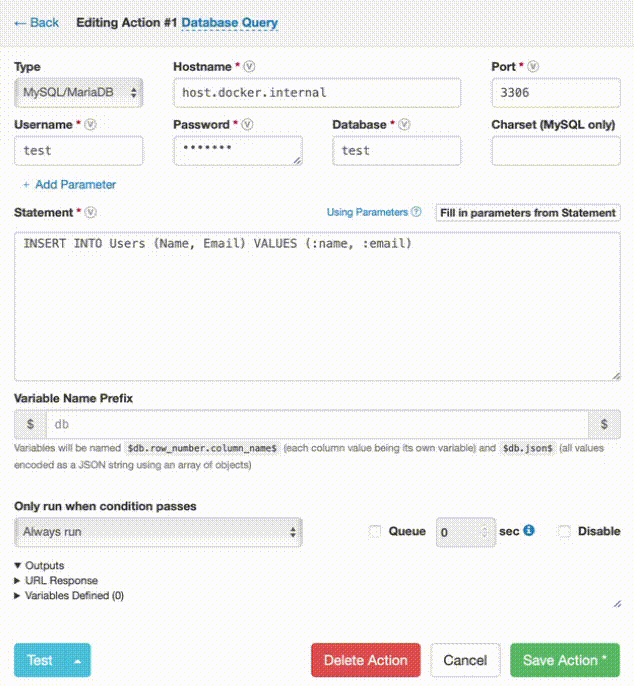
Fetching data¶
When fetching data using e.g. SELECT statements, Webhook.site automatically inserts data in a series of Custom Action Variables, which are then available to downstream actions.
For example, when fetching rows from the following table:
Using the following statement:
If the variable name prefix would be set to output
, the following variables would be created containing specific values:
Variable Name | Value |
---|---|
$output.0.id$ | 1 |
$output.0.fname$ | Simon |
$output.0.lname$ | Fredsted |
$output.0.title$ | Founder |
$output.1.id$ | 2 |
$output.1.fname$ | Jack |
$output.1.lname$ | Daniels |
$output.1.title$ | Assistant |
A variable would be created with the name $output.json$
containing the data in JSON format:
[
{
"id": 1,
"fname": "Simon",
"lname": "Fredsted",
"title": "Founder"
},
{
"id": 2,
"fname": "Jack",
"lname": "Daniels",
"title": "Assistant"
}
]
A variable named $output.rows$
- containing the number of affected rows - would be set to 2
.
If the action encounters an error, the $output.error$
variable is set to the error message. You can use this to check that the action was successful in conjunction with the Conditions action, specifically the variable exists operator.
Postgres Endpoint ID¶
If your database host requires an endpoint ID to be specified, you can do so via the Password field, for example: endpoint=my_endpoint;password
. Replace my_endpoint
with the endpoint ID, and password
with the actual password.
Behavior¶
Don't Save¶
Marks the request so it is not saved in Webhook.site, which is useful (especially in combination with Conditions) when receiving a large amount of requests.
Log¶
Adds a custom log entry to the Request's action output.
Modify Response¶
This action can be used to modify the response of the Webhook.site URL based on the input.
To make a redirect, leave the Response Body field blank, in the Response Headers field enter Location: https://example.com
and set Status Code to 302
.
Rate Limit¶
This action can be used to allow a specific amount of requests in a specific amount of time per a given IP.
If the IP is rate limited, the URL will respond with a HTTP 429
, action execution is stopped, and the request is not saved in Webhook.site.
Stop¶
Immediately stops Custom Action execution and returns the default response.
Basic Auth¶
With this action, the URL is protected with Basic Authentication. If the username and password combination is wrong, the request is automatically marked as Don't Save, action execution is stopped, and the response status is HTTP 401. The action has no effect for emails or DNSHooks.
Basic Auth works with the Authorization HTTP header, where the username and password is encoded in base64, separated by a colon (:
). For example, a successfull request when the username is user
and password is pass123
would have the following header:
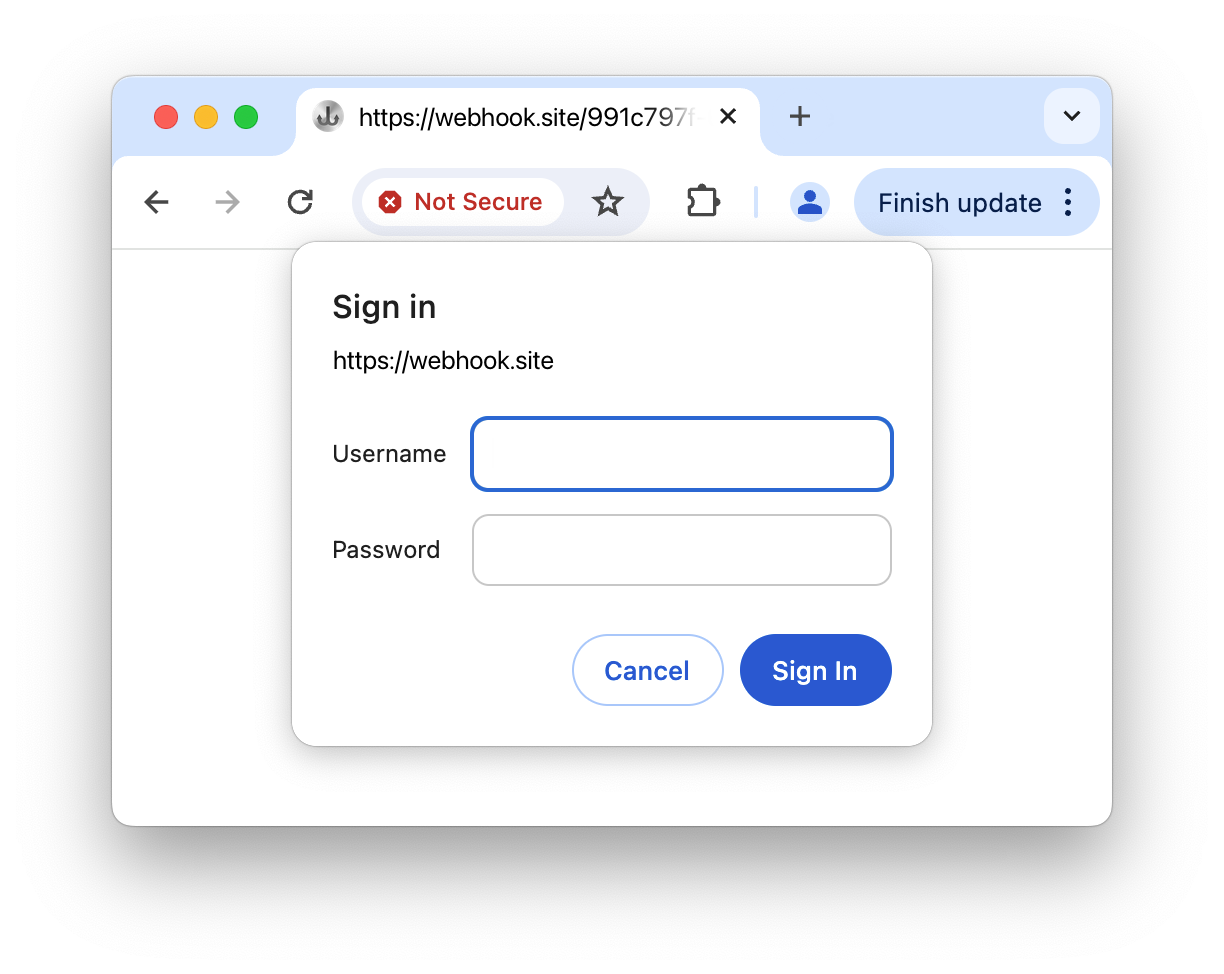
When the URL is visited with a Web browser, a username and password form will be shown.
Mock¶
OpenAPI/Swagger¶
By utilizing the Mock action, you have the ability to upload an OpenAPI or Swagger specification in YAML or JSON, enabling your Webhook.site URL to automatically function as a mock server. This is achieved by dynamically generating responses that align with the paths, endpoints, and data schemas outlined within the uploaded specification, allowing for seamless testing and simulation of API behavior.
If the spec is a URL, Webhook.site will try to download the specification and parse it from JSON or YAML.
An example spec that can be used can be found here.
If your spec defines a server, it's recommended that it's added via the Path field, e.g. https://example.com$request.path$
Video demo
Logic¶
Conditions¶
Useful for validating if variables conform to a set of certain criteria, this action will either create a condition that can be used in downstream actions, or simply stop or continue the action flow entirely.
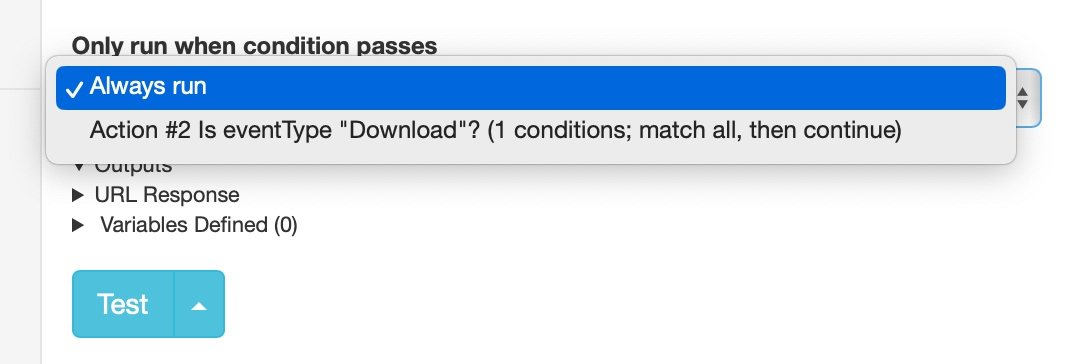
In both the input and the value fields, variables can be used (including Global Variables from the Control Panel), so you can compare e.g. JSONPath or Regex values - or even values from a previous HTTP request that was sent.
Currently, three actions are provided: use result, stop and continue. Use Result allows using the Condition result in downstream actions. Stop will stop further action execution of the condition is a match. Continue will only continue further execution if the condition is a match, and otherwise stop.
Operators with value argument¶
- is equal to
- is not equal to
- starts with
- ends with
- contains
- does not contain
- is greater than
- is greater than or equal to
- is less than
- is less than or equal to
- matches regex – example regex:
/^[a-zA-Z0-9-_]{3,32}$/
Operators without value argument¶
- variable exists - whether a Webhook.site variable is defined
- is true
- is false
- is numeric - whether input is numeric; examples include
42
,123.45
,0x539
,02471
,0b10100111001
- is integer - whether input is an integer;
42
is;123.45
is not. - is float - whether input is a valid float/double (e.g. both
42
and123.45
are valid) - is json - whether input is valid JSON
- is email
- is domain - e.g.
webhook.site
- is URL - e.g.
https://webhook.site?value=xxx
The "result" of the condition will be logged to the action output, so you can see what happened.
Set Variable¶
Defines (or overwrites) a variable that's available to downstream actions. The variable is not saved permanently.
There are several modes:
- Text: Using the default "Text" mode, the variable is simply set to what's entered in the Text field.
- Random String, Random Number: When using the "Random" mode, you can generate a random string for e.g. one-time identifiers and passwords.
- Date: Generate date strings specifying a custom input date and an output format - defaults to ISO-8601 format.
- Math: Easily execute powerful math expressions like
$myvar$ + 1
(increment a Variable) orround($myvar$, 2)
(round to 2 decimals), see below for more
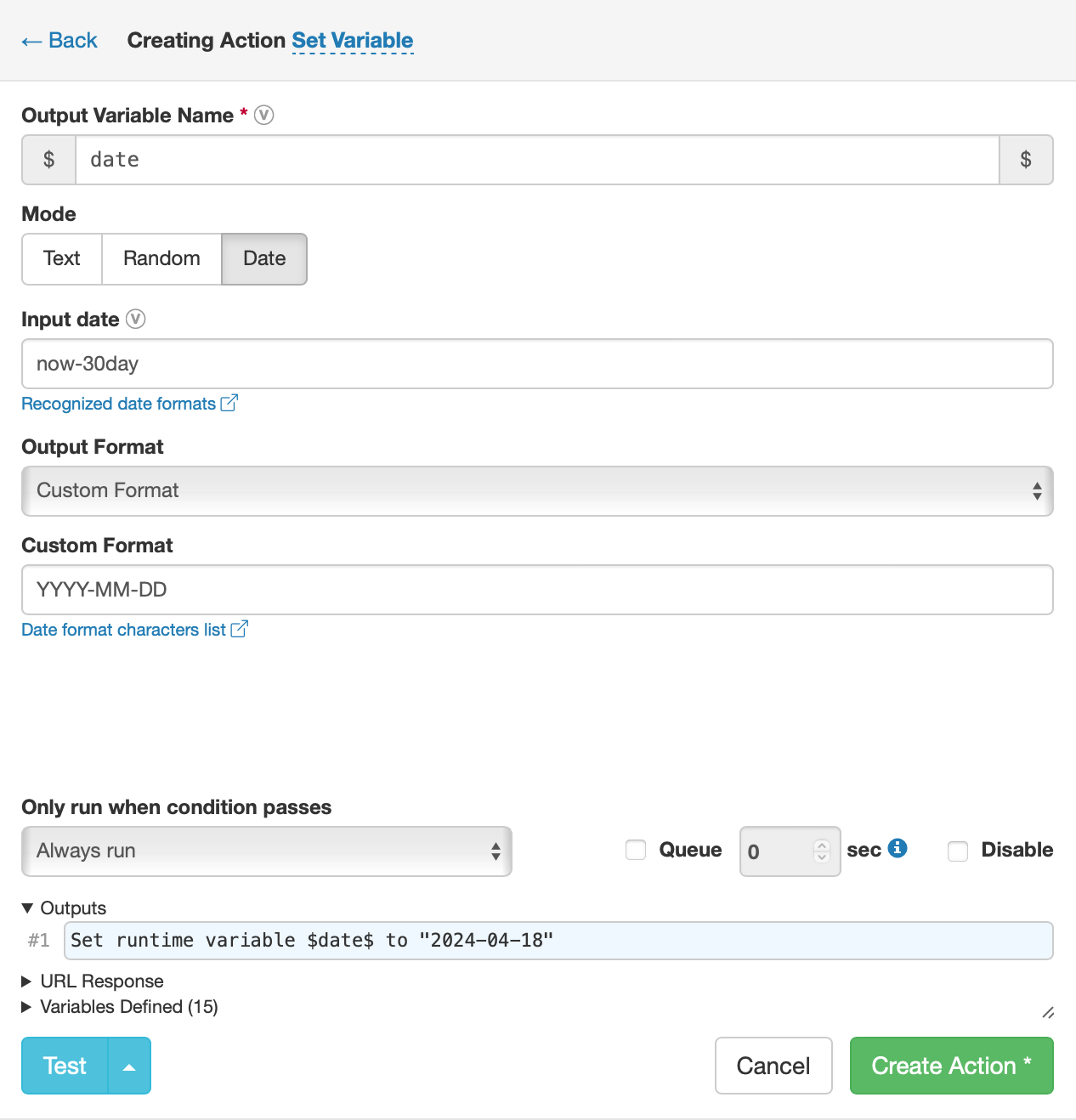
Math Mode¶
Operators supported: + - * / % ^
as well as parentheses ()
and arrays [1, 2, 3]
Supported functions: abs
, acos (arccos)
, acosh
, arccos
, arccosec
, arccot
, arccotan
, arccsc (arccosec)
, arcctg (arccot
, arccotan)
, arcsec
, arcsin
, arctan
, arctg
, array
, asin (arcsin)
, atan (atn
, arctan
, arctg)
, atan2
, atanh
, atn
, avg
, bindec
, ceil
, cos
, cosec
, cosec (csc)
, cosh
, cot
, cotan
, cotg
, csc
, ctg (cot
, cotan
, cotg
, ctn)
, ctn
, decbin
, dechex
, decoct
, deg2rad
, exp
, expm1
, floor
, fmod
, hexdec
, hypot
, if
, intdiv
, lg
, ln
, log (ln)
, log10 (lg)
, log1p
, max
, median
, min
, octdec
, pi
, pow
, rad2deg
, round
, sec
, sin
, sinh
, sqrt
, tan (tn
, tg)
, tanh
, tg
, tn
Functions can accept variable arguments: avg(1, 2, 3)
or arrays: avg([1, 2, 3])
Logical operators (==
, !=
, <
, <
, >=
, <=
, &&
, ||
, !
) are supported, but logically they can only return true (1
) or false (0
). In order to leverage them, use the built in if function: if($a > $b, $a - $b, $b - $a)
Default variables:
$pi
= 3.14159265359$e
= 2.71828182846
Store Global Variable¶
Saves (or overwrites) a Global Variable that's saved permanently and available to all URLs in your account. If you don't need to save the variable permanently, you should use the Set Runtime Variable instead.
Scripting¶
JavaScript¶
With the JavaScript action, you can execute JavaScript code using a Node.js sandbox that runs on the Webhook.site cloud.
General Functions¶
console.log(line)
/ echo(line)
- log a string to Action output
set(variable_name, value)
- sets a Webhook.site variable for use in downstream actions
The following code would set the variable $myvar$ to value
:
get(variable_name)
- gets a Webhook.site variable (except Global Variables; use the global()
function for that)
variables
- global array variable containing Webhook.site variables
global(variable_name)
- retrieves the value of a Webhook.site Global Variable. Must be used async. Returns null
if variable doesn't exist.
store(variable_name, value)
- stores the value of a Webhook.site Global Variable. Must be used async.
stop()
- stops action execution and return response
dont_save()
- marks current requests as Don't Save, so it won't be stored or shown in the Webhook.site requests list
respond(content, status, headers)
- stops action execution and return response
set_response(content, status, headers)
- sets response, but doesn't stop action execution
Utility Modules¶
Code executed with the Webhook.site JavaScript action runs in a sandbox where the following utility libraries are available by using the require()
function:
axios
- HTTP Clientlodash
- General utility librarydayjs
- Date and time manipulationcheerio
- JQuery-like HTML selector libraryjsonpath
- JSONPath query librarycrypto
– Node.js built-in crypto libraryfaker
- Seed data generatornats
- NATS Clientsupabase
- Supabase Clientmoment
- Date and Time libraryform-data
- Form libraryfetch
- HTTP Client
Do you need a library that isn't listed here? Please contact support!
WebhookScript¶
Executes custom scripts using a scripting language that's very similar to JavaScript and PHP.
For more information about WebhookScript, see the dedicated page.
Multimedia¶
Generate PDF¶
Takes either HTML or markdown input and generates a variable ($pdf$
) containing the PDF file contents. You can enter this variable in e.g. an Send Email attachment content field, or with the Modify Response action (remember adding a Content-Type: application/pdf
header!)
If you have issues with special characters/scripts rendering as question marks (?
), make sure you're using a font that supports those characters as the default fonts may not support it. You can import your own fonts using CSS, for example, inserting this HTML snippet in the <head>
section will import the Roboto
font from Google Fonts and use it as the default font:
<link href="https://fonts.googleapis.com/css2?family=Roboto" rel="stylesheet">
<style>* { font-family: Roboto }</style>
Resize Image¶
Takes an image from either a URL or raw image data from e.g. a file upload, email attachment, request response or another action such as Dropbox.
You can enter both width and height to contrain the image in both dimensions, or enter a single dimension.
Check "Keep Aspect Ratio" so that the image keeps the aspect ratio, but doesn't exceed the height and width constraints.
Google Sheets¶
Note
Google Sheets should not be used as a database, and have low usage limits. Google Sheets does not provide a way to query data efficiently. Additionally, if you need to import on the order of thousands of rows or make thousands of calls a day, Google Sheets cannot be used due to rate limits and race conditions. We recommend using a database in conjunction with the Database Query action.
Google Sheets Custom Actions lets you manipulate and retrieve values from a Google Sheet.
The following Google Sheets Custom Actions are available:
- Add Row - appends one or more new rows to an existing spreadsheet
- Update Row - updates one or more cells in an existing spreadsheet
- Get Values - retrieves one or more cell values from an existing spreadsheet
To start, you need to make sure that you have connected a Google account in the Control Panel, available here.
After that, you can select the account in the dropdown when creating the Custom Action.
Usage Limits¶
It is important to note that Google will block Write requests (i.e. adding or updating rows) at 60 requests per minute. After that, the action will temporarily fail with the following error message:
Quota exceeded for quota metric 'Write requests' and limit 'Write requests per minute per user' of service 'sheets.googleapis.com' for consumer
Therefore, for importing mass amounts of data in a short timespan, Google Sheets is not recommended. Instead, we recommend using the Database Query action.
Additionally, Webhook.site will automatically disable Google Sheets actions that continously fail due to e.g. quota errors.
Specifying the spreadsheet¶
When specifying the spreadsheet, you can either just copy/paste the spreadsheet URL or enter the spreadsheet ID. Variables can be used to specify the spreadsheet.
Ranges¶
All actions must specify a range, which behaves similar in all actions. For the Add Row action, Google Sheets will automatically find a "table" (e.g. a homogenous mass of data) and add the values at the bottom.
A range is the same query as in Google Sheets, e.g. to select A1-C3 in Worksheet "Example", enter 'Example'!A1:C3
.
Values¶
When inserting or updating values, you can either enter a value in the text field, or supply multiple cells and/or rows using JSON. To insert two rows, the JSON would be ["cell 1", "cell 2"]
.
Variables¶
The Get Values Action allows you to define variables based on the output. Since this action can return multiple pieces of data, multiple variables are created.
For example, if you select two columns and two rows, e.g. A1:B2
, four variables would be defined:
variable_name.0.0
= value of A1variable_name.0.1
= value of A2variable_name.1.0
= value of B1variable_name.1.1
= value of B2
Additionally, the data is available in JSON, with the variable_name.json
variable being defined, and continuing with the example above, would contain the following JSON:
Microsoft¶
OneDrive¶
With Webhook.site, you can now use your Microsoft account to upload and download files in your OneDrive account using the Upload and Download Custom Actions.
Excel¶
Using the Add Rows and Get Values actions, Webhook.site now allows using your Microsoft account to append and retrieve data from Excel worksheets in your OneDrive account.
Add Rows¶
To use the Add Rows function, the Microsoft API requires that data is inserted into a Table. Therefore, before starting, make sure you have a Table in your workbook, and enter the correct Table name.
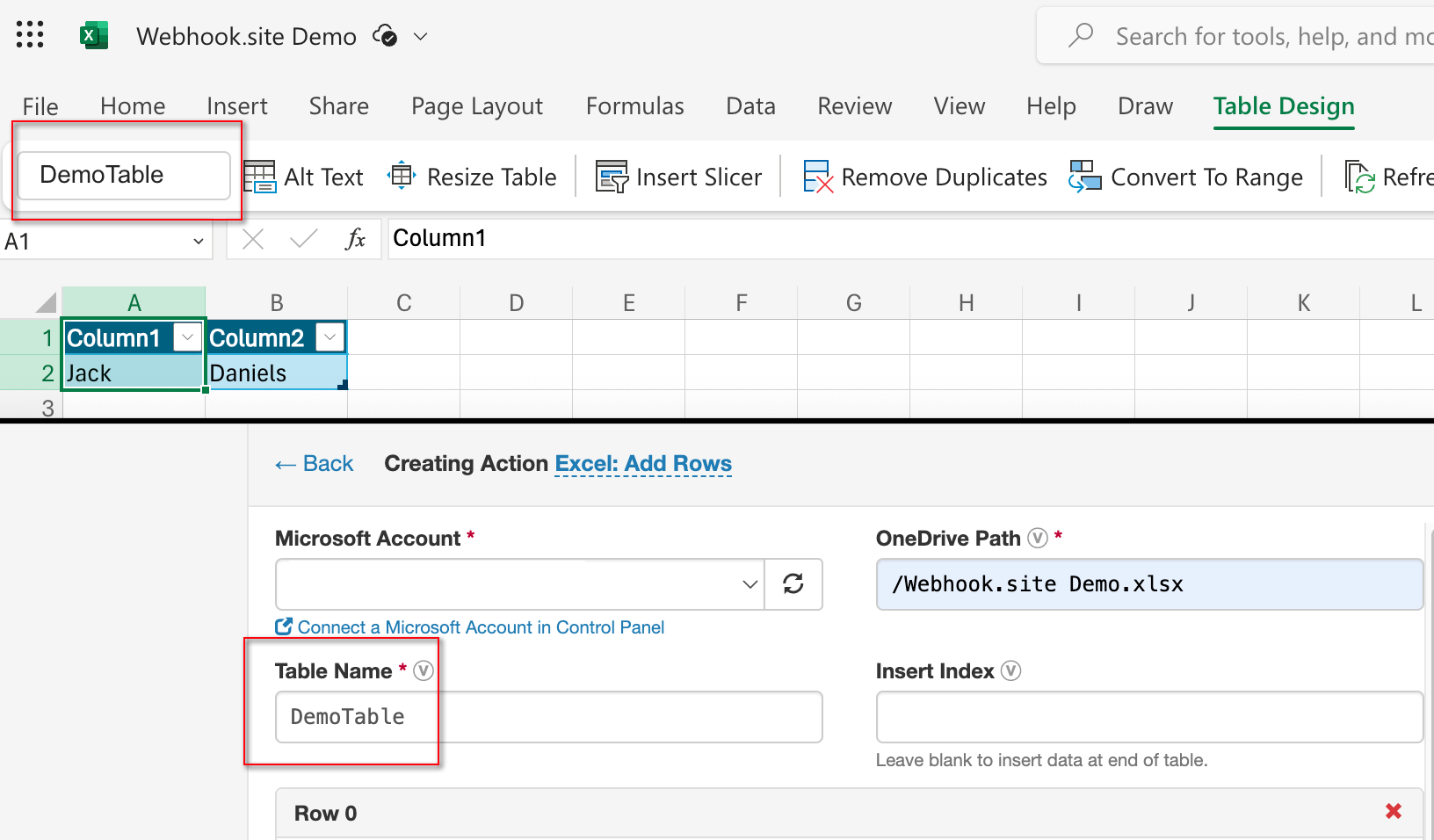
To create a table, select a range and click Insert -> Table.
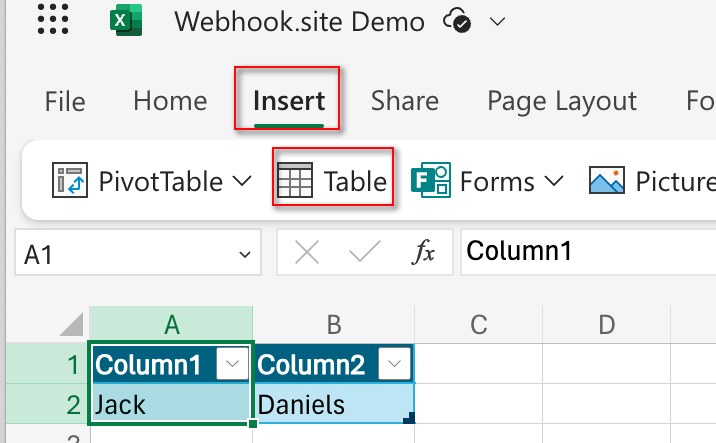
Error: You do not have permissions to open this file in the browser¶
This error seems to have started happening around Feburary 2024 for some users. All 3rd parties - including Webhook.site - that connect to Microsoft's API are affected. There is no known solution at the moment. We encourage users experiencing this to contact Microsoft Support.
Amazon Web Services (AWS)¶
S3¶
The following actions are available for AWS S3:
- Create Bucket
- Create Object
- Delete Object
- Get Object (retrieves object contents to a Variable)
In addition to the "official" Amazon endpoints, Webhook.site also supports S3-compatible storages like Google Cloud Storage, DigitalOcean, MinIO, Wasabi and more. The endpoint can be specified when setting up the account in Control Panel.
Using with Google Cloud Storage¶
To use the S3 action with a Google Cloud Storage bucket, do the following:
- Go to Google Cloud Console → Cloud Storage → Settings → Interoperability
- Create keys for either an existing or a new Google Service Account
- Copy Access key in AWS IAM Key
- Copy Secret in AWS IAM Secret
- Enter
https://storage.googleapis.com
in Custom Endpoint
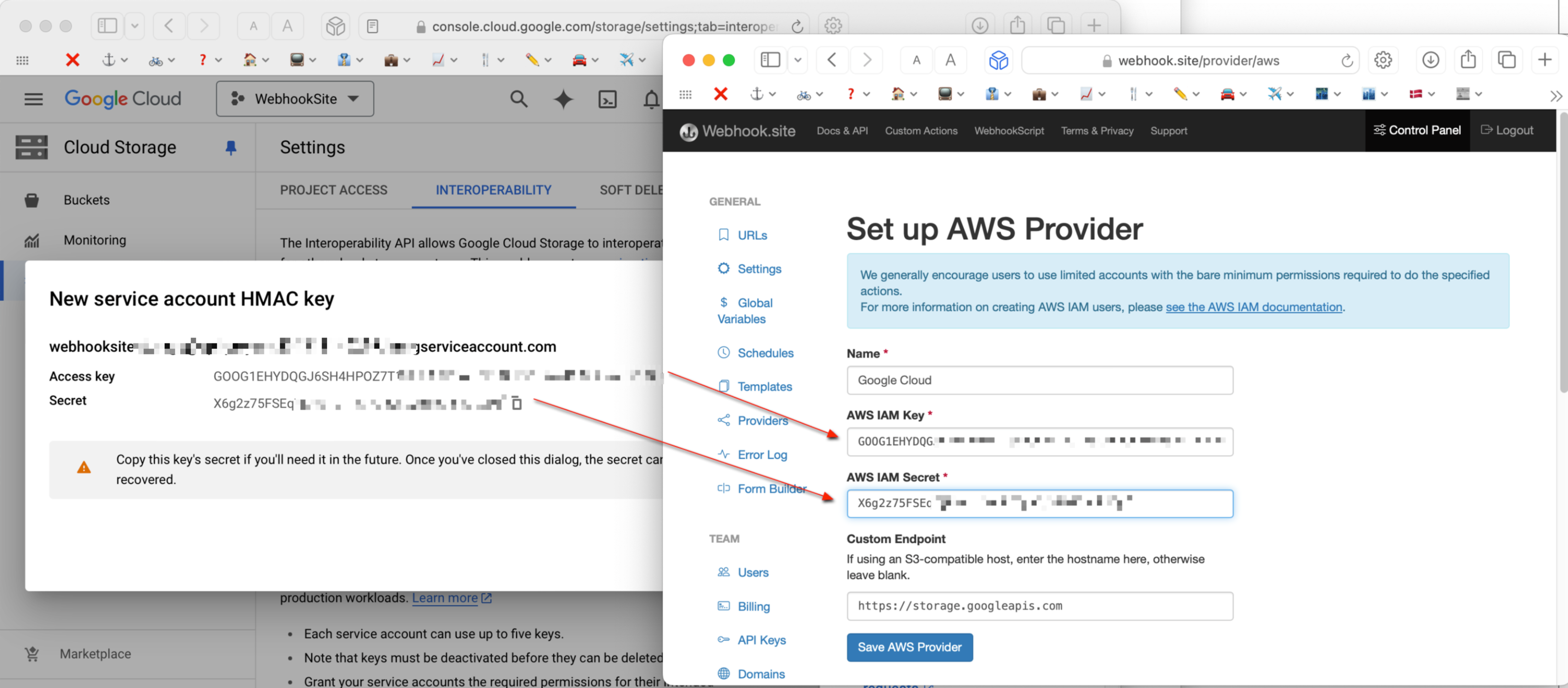
When creating the S3 action, you can leave the Region field blank.
CloudFront¶
The "Create Invalidation" action allows you to dynamically create a CloudFront cache invalidation as a Custom Action. Both the Distribution ID and the paths to be invalidated are replaced with Webhook.site Variables.
Discord¶
With the Discord Custom Action, you can send messages to a specified channel (Each bot account uses a specific channel, so you can connect more accounts to send to different channels or servers.) In addition, you can choose a custom username and avatar image for the bot user.
Slack¶
With the Slack Custom Action, you can easily use Slack's Webhook URLs to send messages to a channel.
Dropbox¶
The Dropbox integration has access to the entire contents of your dropbox, and currently the following actions are available:
- Create Folder
- Download File
- Upload File
- Delete File
- Delete Folder
- Get Link - creates a temporary download link for any file in your Dropbox, and saves it in a variable.
HubSpot¶
With the HubSpot application, you can create Contacts in our HubSpot CRM system. (This action is new, and we're still exploring possibilities - if you want more capabilities for the HubSpot action, please let us know!)
X (formerly known as Twitter)¶
The X Integration supports the following actions using X's API:
- Post Tweet
RabbitMQ¶
The RabbitMQ Integration allows you to publish and consume messages from a RabbitMQ queue by specifying the server connection details.
Pushed¶
With the Send Push Notification action, you can easily send push notifications to your mobile devices using your Pushed.co account.
With a free Pushed.co account, you can send up to 1000 push notifications a month.
ntfy.sh¶
Allows you to easily send push notifications to your browser, phone, watch, etc. Simply download the ntfy.sh app, subscribe to your topic name and send a message to the topic name via this Custom Action. No account required.
App download links: